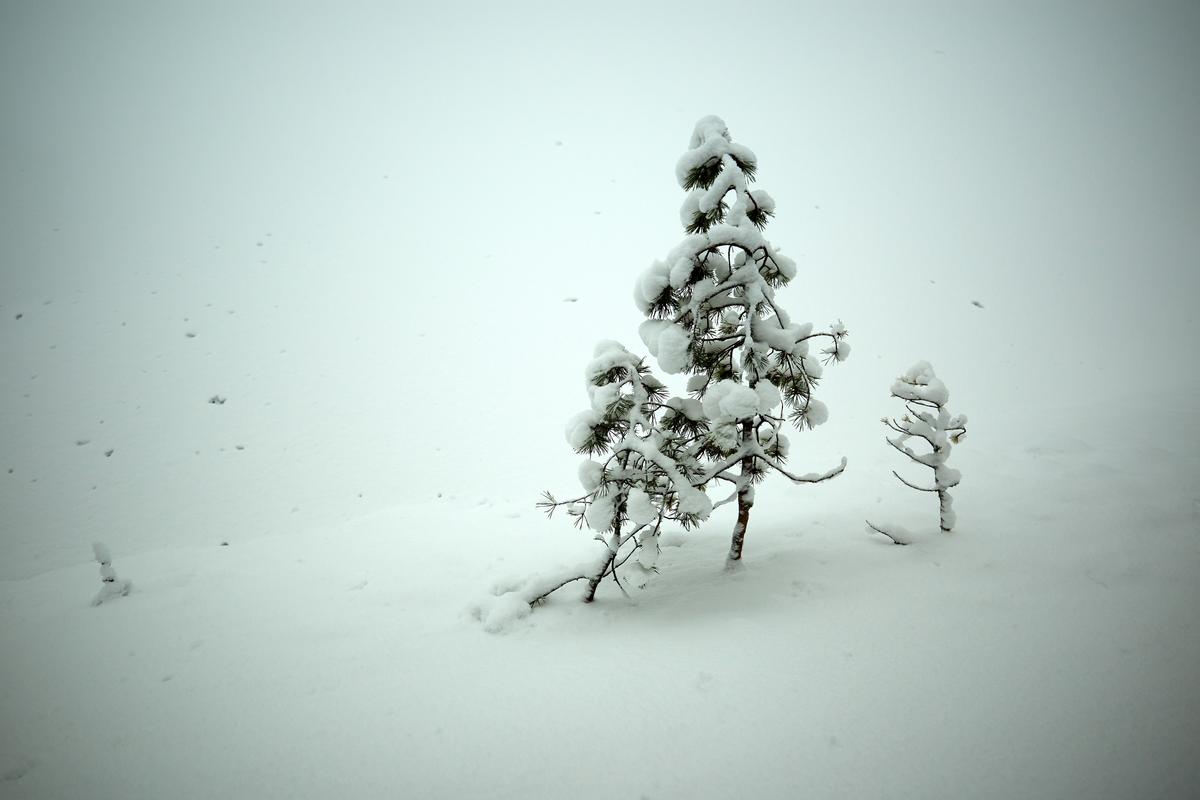
When do you add comments to your code? Do you do it all? Everyone has seen one of these comments:
counter += 1 # increment counter by 1
This comment is explains what is already obvious from the code, so it’s not useful. What’s worse is when somebody then changes the code to be like this:
counter += 2 # increment counter by 1
Now the comment does not match the code anymore. People bring up examples like this when they disparage comments. However, there are other kinds of code comments that are much more valuable.
Explain why the code looks wrong
Code should be written to be easy to understand because understanding is required for both debugging and modifying the code. This is mainly about the code itself: writing clear code, choosing module boundaries carefully, using descriptive names, and maintaining cohesive style.
At times, comments are needed too. In A Philosophy of Software Design, John Ousterhout proposes the following principle:
Comments should describe things that aren’t obvious from the code.
I agree. One heuristic that I’ve lately used is that you should add a comment if the code looks wrong. Think of the next person reading the code – is there anything they would think as weird or out of place? If the wrong-looking code is correct but you can’t make it look correct, you should add a comment.
For example, I recently ran into code that looked like this:
thing1 = create_thing()
thing2 = create_thing()
thing3 = create_thing()
thing4 = create_thing()
for thing in [thing1, thing2, thing3]:
process_thing(thing)
Isn’t there something odd about this? For some reason, thing4
is not processed. Maybe the author of the code has accidentally left it out. Should you go and add it to the list?
In this case, there was a good reason for the omission and the code itself did not need changes. We added a comment, though, explaining the reason right next to the for loop. This way, the next reader will not need to question it.
# `thing4` is not included because it will be processed by another component
for thing in [thing1, thing2, thing3]:
process_thing(thing)
GHC Notes
Sometimes you need to explain the same thing in comments in multiple places. Glasgow Haskell Compiler (GHC) has a nice convention sometimes known as GHC notes. They have a syntax for the long comments that can to be referred to from other places.
My Python adaptation of the style looks like this:
# Note [This is a note]
# ---------------------
# Here goes the long explanation I want to refer to some other place in the code.
# It is usually multiple lines long.
Then you can refer to it from another comment like this:
do_a_thing() # See Note [This is a note]
I’ve used this a bit in the last few months and it feels promising. Now there’s only one place for the information, which makes it easier to keep it up to date.
It would be great to have editor support for this so you could jump to the actual note from the reference. Unfortunately so far I haven’t found anything suitable for VS Code.
Photo: A snow-covered small tree on the shore of Lake Vähä-Parikas.